API design best practices emphasize clarity, adherence to RESTful principles, and comprehensive documentation. They prioritize simplicity, robust error handling, and scalability.
In this guide, you will learn the top 10 API design practices and the stages of API design and find answers to some important questions!
What Is API Design?
API design refers to creating the interface and structure of an Application Programming Interface (API). Hence, it involves defining the endpoints, methods, parameters, data formats, authentication mechanisms, and other technical details that govern how different software systems can interact programmatically.
Good API design is crucial for ensuring that developers can easily understand and use the API. Thus, it meets its intended users’ requirements and remains flexible and scalable as needs evolve.
Effective API design considers usability, consistency, security, performance, and compatibility with existing systems and standards.
Top 10 API Design Best Practices
Effective API design is crucial for building robust, scalable, and developer-friendly APIs to facilitate seamless interactions between software systems.
Here are the top 10 API design best practices
1. Consistency And Predictability
Consistency in API design ensures developers can easily understand and navigate the API, leading to quicker adoption and reduced learning curves.
It uses consistent naming conventions, URL structures, HTTP methods, and response formats across all endpoints. Predictable behavior means that similar actions yield similar results, enhancing the usability and reliability of the API.
2. RESTful Principles
Following REST (Representational State Transfer) principles can help create intuitive, flexible, and scalable APIs.
It includes using resource-based URLs to represent entities and their relationships, employing HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations, and leveraging standard status codes and response formats (such as JSON or XML) for communication.
3. Clear And Comprehensive Documentation
Well-written documentation is essential for guiding developers on how to use the API effectively. It should include detailed descriptions of endpoints, parameters, request and response formats, error-handling procedures, authentication mechanisms, and usage examples.
Providing interactive API documentation tools can enhance the developer experience by allowing for easy exploration and testing of API endpoints.
4. Versioning Strategy
Implementing a versioning strategy enables the API to evolve without breaking backward compatibility with existing clients.
Versioning is achieved through:
- URL paths:
/v1/resource
- Custom headers:
Accept-Version
- Query parameters:
?version=1
Hence, it’s crucial to communicate versioning changes clearly and provide migration paths for deprecated features.
5. Authentication And Authorization
Proper authentication and authorization mechanisms are essential for ensuring the security and integrity of API interactions.
API security can be enforced using standards like OAuth 2.0, API keys, JWT (JSON Web Tokens), or client certificates. Fine-grained authorization controls should also be implemented to restrict access to sensitive resources based on user roles and permissions.
6. Error Handling And Status Codes
Effective error handling is critical for providing meaningful feedback to clients when requests fail or encounter issues.
APIs should use standard HTTP status codes (e.g., 200 OK, 404 Not Found, 500 Internal Server Error) to indicate the success or failure of requests. Error responses should include descriptive error messages, error codes, and guidance on resolving the issue.
7. Pagination And Filtering
APIs that return extensive data collections should support pagination and filtering to improve performance and usability.
Pagination allows clients to retrieve data in manageable chunks, while filtering enables clients to narrow down results based on specific criteria (e.g., date range, category). Standardized pagination parameters (e.g., page, limit) and filter syntax (e.g., ?filter=keyword) should be used for consistency.
8. Optimized Performance
Optimizing API performance is essential for delivering a responsive and efficient user experience. This includes minimizing response times, reducing payload sizes, caching frequently accessed data, and leveraging techniques like gzip compression.
Asynchronous processing and batch operations can improve scalability and throughput for resource-intensive tasks.
9. HATEOAS (Hypermedia as the Engine of Application State)
HATEOAS is a principle that enhances API discoverability and navigability by including hypermedia links in API responses. These links give clients navigational cues to explore related resources and actions dynamically.
By following links provided by the API, clients can interact with the API more intuitively and discover available functionalities without prior knowledge.
10. Feedback And Iteration
Continuous feedback and iteration are essential to refining and improving API design over time. Soliciting input from API consumers, monitoring usage patterns and performance metrics, and iterating based on real-world usage can help identify areas for optimization and enhancement.
Regular updates to documentation, versioning, and backward compatibility ensure that the API remains relevant and effective in meeting evolving user needs.
Stages Of API Design
The process of API design typically involves several stages, each crucial for ensuring that the resulting API meets the requirements of its users and aligns with best practices.
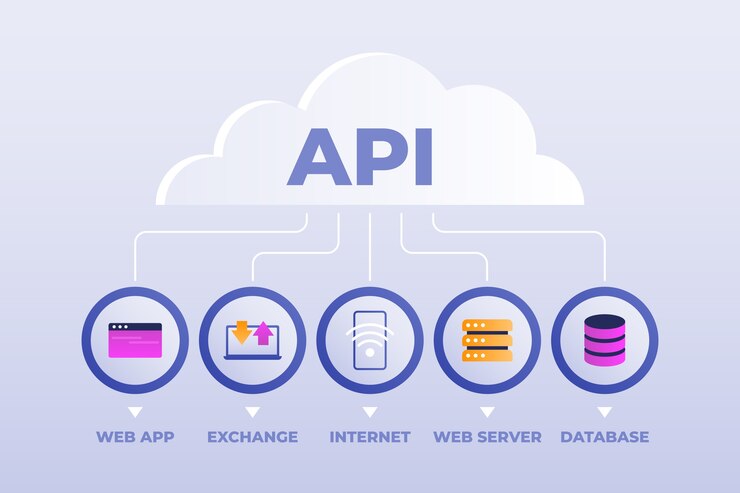
Here are the critical stages of API design.
Requirement Analysis
In this initial stage, the goals and objectives of the API are identified by gathering requirements from stakeholders, including developers, product managers, and end-users.
Key considerations include the intended functionality of the API, the target audience, performance requirements, security needs, integration scenarios, and compatibility with existing systems or platforms.
Use Case Definition
Use cases define the specific interactions and scenarios in which the API will be utilized. Each use case describes a particular action or task the API must support, such as retrieving data, performing calculations, or updating records.
Use cases help clarify the API’s scope and requirements and guide the design decisions throughout the process.
Resource Modeling
Resource modeling involves identifying and defining the entities (resources) the API will expose to clients. Resources represent the data or functionality clients can interact with through the API.
This stage includes defining resource endpoints, attributes, relationships, and the operations (HTTP methods) that can be performed on them.
Data Format And Protocol Selection
The choice of data formats and communication protocols is crucial for ensuring interoperability and compatibility with client applications.
Standard data formats include JSON (JavaScript Object Notation) and XML (eXtensible Markup Language), while popular protocols include HTTP/HTTPS, REST (Representational State Transfer), GraphQL, and SOAP (Simple Object Access Protocol).
Factors such as simplicity, flexibility, performance, and industry standards influence the selection of appropriate formats and protocols.
Designing Endpoint Contracts
Endpoint contracts define the structure and behavior of individual API endpoints, including the URL paths, request and response formats, and supported operations (HTTP methods).
Design decisions in this stage should prioritize clarity, consistency, simplicity, and adherence to RESTful principles. Endpoint contracts should also consider error handling, pagination, filtering, sorting, authentication, authorization, and other cross-cutting concerns.
Security Design
Security design involves identifying and implementing mechanisms to protect the API against common security threats, such as unauthorized access, data breaches, injection attacks, and denial-of-service (DoS) attacks.
This stage includes defining authentication methods, access control policies, encryption requirements, rate limiting, and auditing/logging mechanisms.
Security considerations should be integrated into the API design from the outset to ensure that security controls are built-in rather than added as an afterthought.
Documentation And Specification
Comprehensive documentation and specifications are essential for guiding developers on how to use the API effectively.
This stage involves documenting the API endpoints, parameters, request and response formats, error codes, usage examples, authentication mechanisms, and other relevant information.
API documentation can be provided in various formats, such as OpenAPI (formerly Swagger), RAML (RESTful API Modeling Language), API Blueprint, or custom documentation tools.
Review And Iteration
The API design should undergo thorough review and validation by stakeholders, including developers, architects, product owners, and domain experts.
Review feedback should be incorporated into the design iteratively to address any issues, improve clarity, enhance usability, and ensure alignment with requirements.
Iterative refinement may involve revisiting earlier stages of the design process based on feedback and evolving needs.
Implementation And Testing
Once the API design is finalized, it can be implemented according to the specifications and documentation. Implementation involves writing the code for the API endpoints, data models, business logic, security controls, and other components.
Comprehensive testing is essential to validate the API’s functionality, performance, security, and reliability, including unit tests, integration tests, security assessments, and load testing.
Deployment And Maintenance
After testing and validation, the API is deployed to clients’ production environments.
Ongoing maintenance and support are required to monitor the API’s performance, address issues, apply updates and patches, and accommodate changes in requirements or technology.
Continuous monitoring, logging, versioning, and backward compatibility strategies help ensure the API’s stability, availability, and longevity throughout its lifecycle.
FAQs: API Design Best Practices
How Do You Do Good API Design?
Good API design involves several vital steps to ensure usability and effectiveness. Firstly, it’s essential to clearly define the API’s purpose and functionality to meet users’ needs. Following RESTful principles, such as organizing resources logically and maintaining statelessness. Descriptive resource names, well-defined request/response formats, and thorough documentation enhance understanding and usability.
What Are API Design Principles?
API design principles serve as guiding rules for creating clear, consistent, and user-friendly APIs. These principles include clarity, consistency, and ensuring the API is easy to understand and use. Thus, it helps to maintain uniformity in naming conventions, resource structures, and response formats. Simplicity is vital to keeping the API design straightforward and avoiding unnecessary complexity.
Which Is The Best API Architecture?
Determining the best API architecture depends on various factors, including project requirements, scalability needs, and existing infrastructure. While RESTful architecture is widely adopted for its simplicity, scalability, and compatibility with the HTTP protocol, other architectures such as GraphQL, gRPC, and SOAP may be suitable for specific use cases or environments.
Final Thoughts
In conclusion, API design best practices play a pivotal role in shaping the functionality and usability of APIs in today’s interconnected world. By prioritizing clarity, simplicity, and adherence to standards, developers can craft APIs that meet current requirements and anticipate future needs.
Embracing these best practices enhances the developer experience and contributes to creating robust, interoperable systems that power innovation and drive digital transformation.